First hour of a new Laravel project
0001_01_01_000001_create_cache_table .................................. 1.00ms DONE
0001_01_01_000002_create_jobs_table ................................... 2.08ms DONE
Great! You've just created a new Laravel project and want to do everything right from the start, correct? This guide will walk you through essential steps to optimize your new Laravel project in its first hour.
Configure Eloquent Strictness #
Laravel provides the Model::shouldBeStrict
method that does three things:
- Prevents lazy loading (addressing the N+1 problem). See Preventing Lazy Loading
- Prevents silently discarding attributes (throws an exception when attempting to fill an unfillable attribute)
- Prevents accessing missing attributes
The "lazy loading" prevention often depends on database data, so it's better to avoid throwing an exception in production. For the other two, you can enable them in all environments:
// AppServiceProvider.php or any other service provider
public function boot(): void
{
Model::preventLazyLoading(! $this->app->isProduction());
Model::preventSilentlyDiscardingAttributes();
Model::preventAccessingMissingAttributes();
}
Choose Primary Key Type #
There are three common types of primary keys:
- Auto-incrementing integer (technically, by default it's an
UNSIGNED BIGINT
) - UUID (example:
6e48762e-8c54-43ab-b2f2-09eebb59ad9b
) - ULID (example:
01j2qxp0rg5618brj9c9kmrk6h
)
Choosing the right type is a big topic (you can start by reading the Laravel documentation).
If you choose UUID or ULID, you can instruct Laravel to use the proper morph key type
when you use $table->morphs()
and $table->nullableMorphs()
in migrations:
// AppServiceProvider.php or any other service provider
public function boot(): void
{
Builder::morphUsingUuids(); // for UUID
Builder::morphUsingUlids(); // for ULID
}
Other packages may (and should) also respect this setting (e.g., Laravel Nova).
Enforce Morph Map #
If you plan to rename your Models (rename a class or change a namespace),
I recommend enforcing a morph map to avoid data corruption.
This way, Laravel will not store the fully qualified class name (FQCN) in the database, but a short alias instead.
If an alias is not set, Laravel will throw a \Illuminate\Database\ClassMorphViolationException
exception.
// AppServiceProvider.php or any other service provider
public function boot(): void
{
Relation::enforceMorphMap([
'user' => User::class,
'team' => Team::class,
]);
}
Install Composer Packages #
Clockwork #
In my opinion, Clockwork is the best tool of the "Laravel debug bar" type, as it doesn't change your HTML output.
composer require itsgoingd/clockwork --dev
Larastan #
Larastan is an essential static analysis tool for Laravel projects. With a minimal time investment, you can get a great reporting tool for potential bugs.
composer require larastan/larastan --dev
Paratest #
Paratest enables parallel testing for PHPUnit/Pest, which will make your tests run faster (proportional to the number of cores in your CPU).
composer require brianium/paratest --dev
After installation, please check the official Laravel documentation on Running Tests in Parallel.
Composer Dependency Analyser #
Composer Dependency Analyser provides fast detection of composer dependency issues such as:
- Unused dependencies
- Shadow dependencies
- Misplaced dependencies
- Dev dependency on production code
- Production dependency that is only in dev code
composer require --dev shipmonk/composer-dependency-analyser
Set up a CI/CD Pipeline #
Set up a CI/CD pipeline with GitHub Actions, GitLab CI, or any other CI/CD service. I've prepared a simple Laravel GitHub workflows starting pack that includes three workflows:
- Main (runs tests, security checks, and deploys)
- Code quality (non-blocking deployment)
- Code style check (non-blocking deployment)
Document Your Project #
Create a comprehensive README.md and list all commands needed to run, test, develop, and deploy the project. Even if you're the only developer, your future self will thank you for this thorough documentation.
Conclusion #
Since we're limited to one hour, I'll conclude here. These initial steps will set a solid foundation for your development process. There are many more ways to improve your Laravel project, most of which are project-specific.
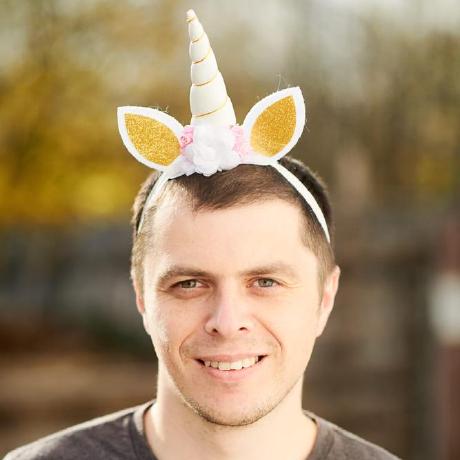
- Previous: sscanf simplifies your regex