sscanf simplifies your regex
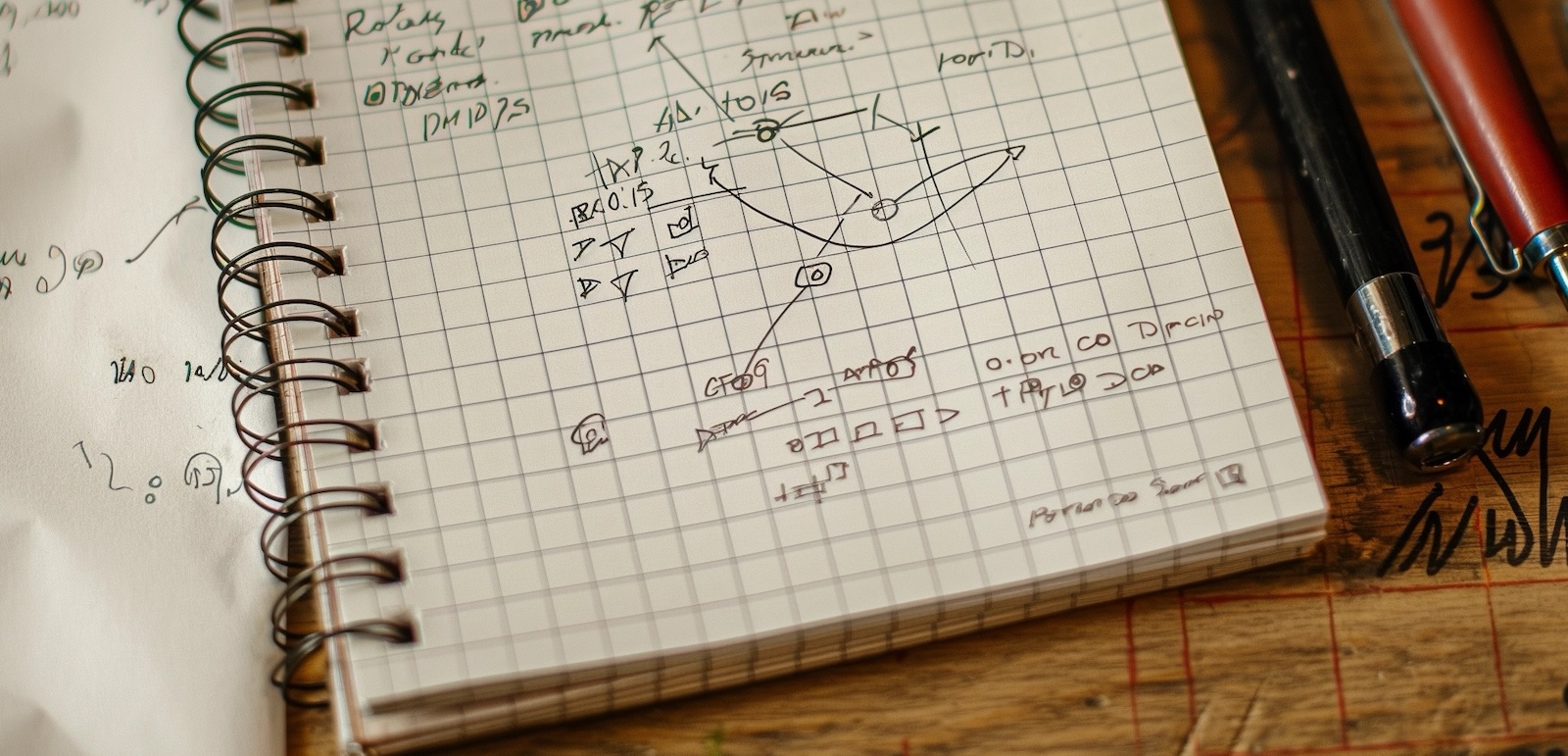
The sscanf
PHP function offers a powerful alternative to regular expression functions like preg_match
for parsing strings.
While less flexible than regex, sscanf
can be more readable and efficient for simple parsing tasks.
Surprisingly, sscanf
is a hidden gem in PHP, and many developers are unaware of its existence.
It's a wrapper around the C library function, and it's available in PHP since the early days.
Basic examples #
Example 1: Basic usage
$input = 'John 25 New York';
sscanf($input, '%s %d %s', $name, $age, $city);
Example 2: Parsing a date string
$date = '2023-07-08';
sscanf($date, '%d-%d-%d', $year, $month, $day);
Example 3: Parsing a complex log entry
$logEntry = "2023-07-08 14:30:45 [WARNING] User authentication failed: username=johndoe, ip=192.168.1.100";
$pattern = "%d-%d-%d %d:%d:%d [%s] %[^:]: username=%[^,], ip=%s";
sscanf($logEntry, $pattern, $year, $month, $day, $hour, $minute, $second, $level, $message, $username, $ip);
sscanf vs preg_match #
Parsing a URL example, diff:
-preg_match('/^(\s+):\/\/([^\/]+)\/(.*)$/', $url, $matches);
-$scheme = $matches[1];
-$domain = $matches[2];
-$path = $matches[3];
+sscanf($url, "%[^:]://%[^/]/%s", $scheme, $domain, $path);
4 lines vs. 1 line!!
Of course, it’s a simplified example (for real URL parsing, please use parse_url
), but shares the idea.
While sscanf
isn't a complete replacement for regex, it can simplify many common string parsing tasks in PHP.
Still need to write a regex? Check out a great Writing better Regular Expressions in PHP article.
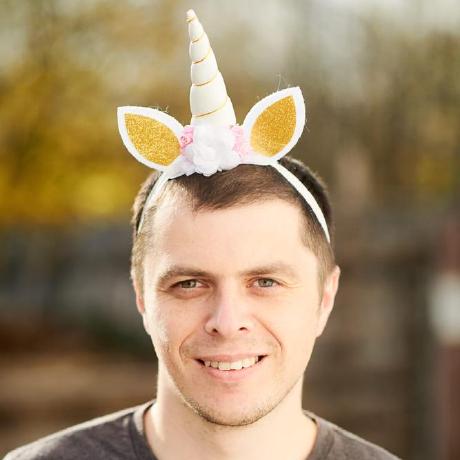